Things we will proceed with:
1. edit Functions.php
2. Create featured-image-for-categories.php file in theme or child theme
3. Two placeholder Images inside theme folder: placeholder.png and placeholder-thumb.png
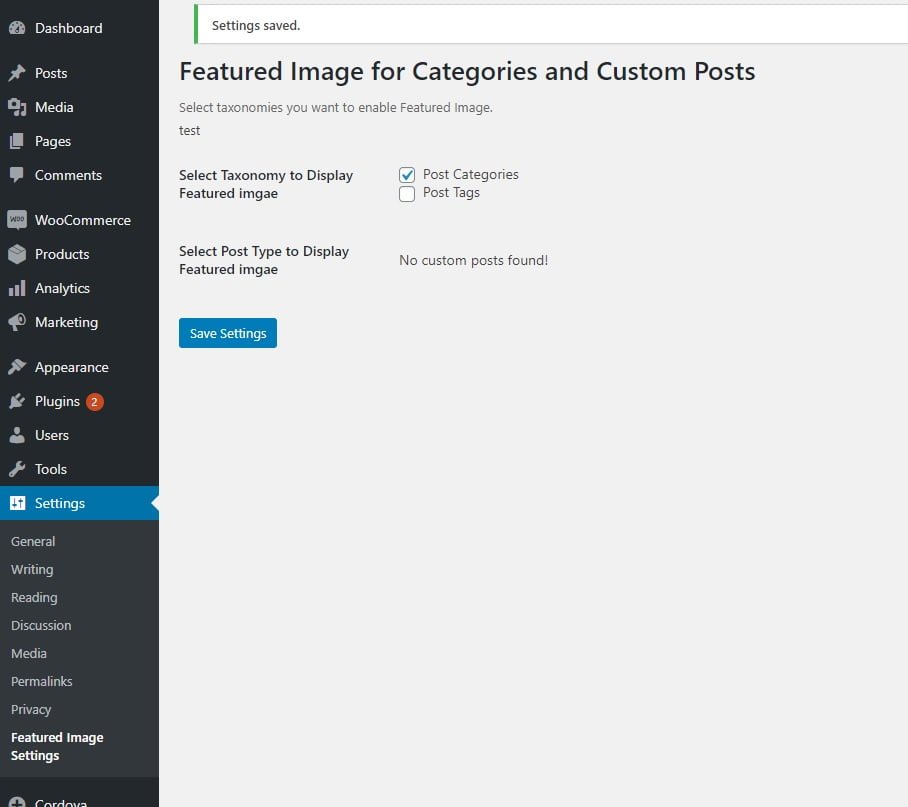
Step1. Add this code to functions.php file
include('featured-image-for-categories.php');
new WP_Simple_Featured_Image;
Step2 : Create a new file with name “featured-image-for-categories.php” and add the below code to this file.
<?php
if (!defined('ABSPATH')) {
exit; // Exit if accessed directly
}
class WP_Simple_Featured_Image{
public $wpsfi_taxonomy = array();
public function __construct(){
$wpsfi_taxonomy = get_option( 'wpsfi_taxonomy' );
$wpsfi_post_type = get_option( 'wpsfi_post_type' );
$this->wpsfi_taxonomy = $wpsfi_taxonomy;
if( !empty( $wpsfi_taxonomy ) ){
foreach( $wpsfi_taxonomy as $taxonomy ){
add_action( $taxonomy.'_add_form_fields', array( $this, 'featured_taxonomy_field' ) );
add_action( $taxonomy.'_edit_form_fields', array( $this, 'edit_featured_taxonomy_field' ) );
// manage_edit-product_cat_columns
add_filter('manage_edit-'.$taxonomy.'_columns', array( $this, 'taxonomy_columns_header' ) );
add_filter('manage_'.$taxonomy.'_custom_column', array( $this, 'taxonomy_columns_content_taxonomy' ), 10, 3 );
}
}
if( !empty( $wpsfi_post_type ) ){
foreach ( $wpsfi_post_type as $post_type ) {
add_filter('manage_edit-'.$post_type.'_columns', array( $this, 'post_columns_header' ) );
add_filter('manage_'.$post_type.'_posts_custom_column', array( $this, 'post_columns_content' ), 10, 2 );
}
}
add_filter('manage_edit-post_columns', array( $this, 'post_columns_header' ) );
add_filter('manage_posts_custom_column', array( $this, 'post_columns_content' ), 10, 2 );
add_action( 'created_term', array( $this, 'save_wpsfi_taxonomy_fields' ), 10, 3 );
add_action( 'edit_term', array( $this, 'save_wpsfi_taxonomy_fields' ), 10, 3 );
add_action('admin_menu', array( $this, 'settings_menu' ) );
add_action( 'admin_init', array( $this, 'register_setting_options' ) );
add_action( 'admin_enqueue_scripts', array( $this, 'admin_scripts' ) );
}
public function admin_scripts(){
wp_enqueue_media();
}
public function register_setting_options(){
register_setting( 'wpsfi_settings_group', 'wpsfi_taxonomy' );
register_setting( 'wpsfi_settings_group', 'wpsfi_post_type' );
}
public function settings_menu(){
add_submenu_page(
'options-general.php',
__( 'Featured Image Settings', 'wp-simple-featured-image' ),
__( 'Featured Image Settings', 'wp-simple-featured-image' ),
'manage_options',
'wpsfi-settings',
array( $this, 'settings_menu_callback' )
);
}
function save_wpsfi_taxonomy_fields( $term_id, $tt_id = '', $taxonomy = '' ){
$wpsfi_taxonomy = $this->wpsfi_taxonomy;
if( empty( $wpsfi_taxonomy ) ){
$wpsfi_taxonomy = array();
}
if ( isset( $_POST['wpsfi_tax_image_id'] ) && in_array( $taxonomy, $wpsfi_taxonomy ) ) {
update_term_meta( $term_id, 'wpsfi_tax_image_id', $_POST['wpsfi_tax_image_id'] );
}
}
public function settings_menu_callback(){
?>
<h1><?php _e('Featured Image for Categories and Custom Posts', 'wp-simple-featured-image' ); ?></h1>
<p class="description"><?php _e('Select taxonomies you want to enable Featured Image.', 'wp-simple-featured-image' ); ?></p>
<form method="post" action="options.php">
<?php
echo get_option( 'wpsfi_post_type' ).'test';
settings_fields( 'wpsfi_settings_group' );
do_settings_sections( 'wpsfi_settings_group' );
$wpsfi_taxonomy = get_option( 'wpsfi_taxonomy' ) ? get_option( 'wpsfi_taxonomy' ) : array() ;
$wpsfi_post_type = get_option( 'wpsfi_post_type' ) ? get_option( 'wpsfi_post_type' ) : array() ;
$registered_taxonomies = wpsfi_get_registered_taxonomy();
$post_type_list = wpsfi_post_type_list();
?>
<table class="form-table">
<tr>
<th><?php _e('Select Taxonomy to Display Featured imgae', 'wp-simple-featured-image' ); ?></th>
<td>
<?php if( !empty( $registered_taxonomies ) ): foreach( $registered_taxonomies as $key => $value): ?>
<input type="checkbox" name="wpsfi_taxonomy[]" value="<?php echo $key; ?>" <?php echo ( in_array( $key, $wpsfi_taxonomy ) ) ? 'checked' : '' ; ?>> <?php echo $value; ?><br/>
<?php endforeach; endif; ?>
</td>
</tr>
<tr>
<th><?php _e('Select Post Type to Display Featured imgae', 'wp-simple-featured-image' ); ?></th>
<td>
<?php if( !empty( $post_type_list ) ): foreach( $post_type_list as $postType): ?>
<input type="checkbox" name="wpsfi_post_type[]" value="<?php echo $postType; ?>" <?php echo ( in_array( $postType, $wpsfi_post_type ) ) ? 'checked' : '' ; ?>> <?php echo $postType; ?><br/>
<?php
endforeach;
else:
echo 'No custom posts found!';
endif; ?>
</td>
</tr>
</table>
<?php submit_button( __( 'Save Settings', 'wp-simple-featured-image' ) ); ?>
</form>
<?php
}
function taxonomy_columns_header( $defaults ){
$defaults['wpsfi_featured_image'] = __('Featured Image', 'wp-simple-featured-image' );
return $defaults;
}
function taxonomy_columns_content_taxonomy( $columns, $column, $id ){
if ( 'wpsfi_featured_image' === $column ) {
$imageID = get_term_meta( $id, 'wpsfi_tax_image_id', true );
$image_url = wpsfi_get_featured_image_url( $id );
$columns .= '<img data-id="'.$imageID.'" src="' . esc_url( $image_url ) . '" alt="' . esc_attr__( 'Thumbnail', 'wp-simple-featured-image' ) . '" class="wpsfi-image" height="48" width="48" />';
}
return $columns;
}
function post_columns_header( $defaults ){
$defaults['wpsfi_featured_image'] = __('Featured Image', 'wp-simple-featured-image' );
return $defaults;
}
function post_columns_content( $column, $post_id ){
if ( 'wpsfi_featured_image' === $column ) {
if( has_post_thumbnail( $post_id ) ){
echo get_the_post_thumbnail( $post_id, array( 48, 48) );
}else{
echo '<img src="'.wpsfi_placeholder_image(false).'" alt="Thumbnail" class="wpsfi-image" height="48" width="48">';
}
}
}
public function featured_taxonomy_field(){
?>
<div class="form-field wpsfi_taxonomy_featured_image">
<label><?php _e( 'Featured Image', 'wp-simple-featured-image' ); ?></label>
<div id="wpsfi_tax_image" style="float: left; margin-right: 10px;"><img src="<?php echo esc_url( wpsfi_placeholder_image() ); ?>" width="60px" height="60px" /></div>
<div style="line-height: 60px;">
<input type="hidden" id="wpsfi_tax_image_id" name="wpsfi_tax_image_id" />
<button type="button" class="upload_image_button button"><?php _e( 'Upload/Add image', 'wp-simple-featured-image' ); ?></button>
<button type="button" class="remove_image_button button"><?php _e( 'Remove image', 'wp-simple-featured-image' ); ?></button>
</div>
<script type="text/javascript">
jQuery(document).ready( function($){
if ( ! $( '#product_cat_thumbnail_id' ).val() ) {
$( '.remove_image_button' ).hide();
}
var file_frame;
$( 'body' ).on( 'click', '.upload_image_button', function( event ) {
event.preventDefault();
if ( file_frame ) {
file_frame.open();
return;
}
file_frame = wp.media.frames.downloadable_file = wp.media({
title: '<?php _e( 'Choose a Fetured Image', 'wp-simple-featured-image' ); ?>',
button: {
text: '<?php _e( 'Use as Fetured Image', 'wp-simple-featured-image' ); ?>'
},
multiple: false
});
file_frame.on( 'select', function() {
var attachment = file_frame.state().get( 'selection' ).first().toJSON();
var attachment_thumbnail = attachment.sizes.thumbnail || attachment.sizes.full;
$( '#wpsfi_tax_image_id' ).val( attachment.id );
$( '#wpsfi_tax_image' ).find( 'img' ).attr( 'src', attachment_thumbnail.url );
$( '.remove_image_button' ).show();
$( '.upload_image_button' ).hide();
});
file_frame.open();
});
$( 'body' ).on( 'click', '.remove_image_button', function() {
$( '#wpsfi_tax_image' ).find( 'img' ).attr( 'src', '<?php echo esc_js( wpsfi_placeholder_image() ); ?>' );
$( '#wpsfi_tax_image_id' ).val( '' );
$( '.remove_image_button' ).hide();
$( '.upload_image_button' ).show();
return false;
});
$( 'body' ).ajaxComplete( function( event, request, options ) {
if ( request && 4 === request.readyState && 200 === request.status
&& options.data && 0 <= options.data.indexOf( 'action=add-tag' ) ) {
var res = wpAjax.parseAjaxResponse( request.responseXML, 'ajax-response' );
if ( ! res || res.errors ) {
return;
}
$( '#wpsfi_tax_image' ).find( 'img' ).attr( 'src', '<?php echo esc_js( wpsfi_placeholder_image() ); ?>' );
$( '#wpsfi_tax_image_id' ).val( '' );
return;
}
} );
});
</script>
<div style="clear:both;"></div>
</div>
<?php
}
public function edit_featured_taxonomy_field( $term ){
$thumbnail_id = get_term_meta( $term->term_id, 'wpsfi_tax_image_id', true );
if ( $thumbnail_id ) {
$image = wp_get_attachment_thumb_url( $thumbnail_id );
} else {
$image = wpsfi_placeholder_image();
}
?>
<tr class="form-field">
<th scope="row" valign="top"><label><?php _e( 'Featured Image', 'wp-simple-featured-image' ); ?></label></th>
<td>
<div id="wpsfi_tax_image" style="float: left; margin-right: 10px;"><img src="<?php echo esc_url( $image ); ?>" width="60px" height="60px" /></div>
<div style="line-height: 60px;">
<input type="hidden" id="wpsfi_tax_image_id" name="wpsfi_tax_image_id" value="<?php echo $thumbnail_id; ?>" />
<button type="button" class="upload_image_button button"><?php _e( 'Upload/Add image', 'wp-simple-featured-image' ); ?></button>
<button type="button" class="remove_image_button button"><?php _e( 'Remove image', 'wp-simple-featured-image' ); ?></button>
</div>
<script type="text/javascript">
jQuery(document).ready( function($){
var tax_thumbnail = $( '#wpsfi_tax_image' ).val();
if ( '0' === tax_thumbnail || '' === tax_thumbnail ) {
$( '.remove_image_button' ).hide();
}
var file_frame;
$( 'body' ).on( 'click', '.upload_image_button', function( event ) {
event.preventDefault();
if ( file_frame ) {
file_frame.open();
return;
}
file_frame = wp.media.frames.downloadable_file = wp.media({
title: '<?php _e( 'Choose a Fetured Image', 'wp-simple-featured-image' ); ?>',
button: {
text: '<?php _e( 'Use as Fetured Image', 'wp-simple-featured-image' ); ?>'
},
multiple: false
});
file_frame.on( 'select', function() {
var attachment = file_frame.state().get( 'selection' ).first().toJSON();
var attachment_thumbnail = attachment.sizes.thumbnail || attachment.sizes.full;
$( '#wpsfi_tax_image_id' ).val( attachment.id );
$( '#wpsfi_tax_image' ).find( 'img' ).attr( 'src', attachment_thumbnail.url );
$( '.remove_image_button' ).show();
$( '.upload_image_button' ).hide();
});
file_frame.open();
});
$( 'body' ).on( 'click', '.remove_image_button', function() {
$( '#wpsfi_tax_image' ).find( 'img' ).attr( 'src', '<?php echo esc_js( wpsfi_placeholder_image() ); ?>' );
$( '#wpsfi_tax_image_id' ).val( '' );
$( '.remove_image_button' ).hide();
$( '.upload_image_button' ).show();
return false;
});
});
</script>
<div style="clear:both;"></div>
</td>
</tr>
<?php
}
}
/* ++++++++++++++++++++++++ Display +++++++++++++++++++++++++*/
function wpsfi_placeholder_image( $default = true ){
if( $default ){
return get_stylesheet_directory_uri().'/placeholder.png';
}else{
return get_stylesheet_directory_uri().'/placeholder-thumb.png';
}
}
function wpsfi_get_featured_image_url( $termID, $placeholderSize = true, $size = false ){
$imageID = get_term_meta( $termID, 'wpsfi_tax_image_id', true );
if ( $imageID ) {
$image_url = wp_get_attachment_thumb_url( $imageID );
if( $size ){
$image_url = wp_get_attachment_image_url( $imageID, $size );
}
} else {
$image_url = wpsfi_placeholder_image( $placeholderSize );
}
$image_url = str_replace( ' ', '%20', $image_url );
return $image_url;
}
/*
You can display the Taxonomy Featured Image in the front end using php function.
wpsfi_display_image( $termID, $size = “medium”, $class = ”, $width = ”, $height =” )
Parameters:
* $termID – (Integer) Taxonomy termID
* $size – (String) Image size
* $class – (String) Add custom class
* $width – (Integer) Width in px.
* $height – (Integer) Height in px
*/
function wpsfi_display_image( $termID, $size = "medium", $class = '', $width = '', $height ='', $placeholderSize = true ){
$imageID = get_term_meta( $termID, 'wpsfi_tax_image_id', true );
$image = '<img class="wpsfi-featured-image" src="'.wpsfi_placeholder_image( $placeholderSize ).'" alt="placeholder"';
if( $imageID ){
if( $width && $height ){
$image = wp_get_attachment_image( $imageID, array( $width, $height ), false, array( "class" => 'wpsfi-featured-image '.$class ) );
}else{
$image = wp_get_attachment_image( $imageID, $size, false, array( "class" => 'wpsfi-featured-image '.$class ) );
}
}
return $image;
}
function wpsfi_get_registered_taxonomy(){
$taxonomies = array();
$args = array(
'public' => true,
'publicly_queryable' => true,
'exclude_from_search' => false
);
$post_types = get_post_types( $args );
if( !empty( $post_types ) ){
foreach( $post_types as $key => $value ){
// Exclude Woocommerce taxonomy
if( $key == 'product' ){
continue;
}
$object_taxonomies = get_object_taxonomies( $key, 'objects' );
foreach( $object_taxonomies as $tax => $obj_tax ){
if( $obj_tax->show_ui ){
$taxonomies[$tax] = ucfirst( $key ).' '.$obj_tax->label;
}
}
}
}
return $taxonomies;
}
function wpsfi_excluded_post_type_list(){
return apply_filters( 'wpsfi_excluded_post_type', array(
'product', 'product_variation', 'shop_order', 'shop_order_refund', 'shop_coupon'
) );
}
function wpsfi_post_type_list(){
$post_type_list = array();
$post_types = get_post_types( array( '_builtin' => false ) );
if( !empty( $post_types ) ){
foreach ($post_types as $key => $value ) {
if( in_array( $key, wpsfi_excluded_post_type_list() ) ){
continue;
}
$post_type_list[] = $key;
}
}
return $post_type_list;
}
// Enable all Custom Post Type display Featured Image
add_action( 'admin_head', function(){
global $wpdb;
$wpsfi_post_type = $wpdb->get_var( "SELECT count(*) FROM `{$wpdb->prefix}options` WHERE `option_name` LIKE 'wpsfi_post_type' LIMIT 1" );
if( !$wpsfi_post_type ){
update_option( 'wpsfi_post_type', wpsfi_post_type_list() );
}
} );
Step 3: Download the Placeholder images and add to your themes directory.
placeholder.png and placeholder-thumb.png
Step 4: To show the images on frontend use this code help in category.php file or make a shortcode for category template.
You can display the Taxonomy Featured Image in the front end using php function.
/* get category id on category template */
$category = get_queried_object();
$termID = $category->term_id;
wpsfi_display_image( $termID, $size = “medium”, $class = ”, $width = ”, $height =” )
Parameters:
* $termID – (Integer) Taxonomy termID
* $size – (String) Image size
* $class – (String) Add custom class
* $width – (Integer) Width in px.
* $height – (Integer) Height in px
Done!